페이지 간 이동을 할 때, Web과 달리, React Native에서는 Stack 구조로 되어있는 React Navigation을 사용한다.
Web에서는 url을 통해 라우팅을 했다면, RN에서는 화면이 기존의 화면 위에 계속해서 쌓이게 된다.
React Navigation을 사용하기 위해
npm install @react-navigation/native
npm install react-native-reanimated react-native-gesture-handler react-native-screens react-native-safe-area-context @react-native-community/masked-view
npm install @react-navigation/stack
다음 명령어를 사용해 패키지를 설치해준다.
npx pod-install ios
cocoapods가 설치되어 있지 않다면, cocoapods도 설치해준다.
다음으로 React Navigation을 사용하기 위해
import { NavigationContainer } from '@react-navigation/native';
import { createNativeStackNavigator } from '@react-navigation/native-stack';
NavagationContainer와 createNativeStackNavigator를 import 해준다.
NavagationContainer로 최상단에서 감싸주어야 하며, createNativeStackNavigator를 통해 Navigation Stack을 생성해줄 수 있다.
// In App.js in a new project
import * as React from 'react';
import { View, Text } from 'react-native';
import { NavigationContainer } from '@react-navigation/native';
import { createNativeStackNavigator } from '@react-navigation/native-stack';
function HomeScreen() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
</View>
);
}
const Stack = createNativeStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
위와 같이 Stack을 생성하고 Screen을 나눠줄 수 있다. 최상단에서 NavigationContainer로 감싸준다.
처음 app을 실행했을 때 보여지는 스크린은 Stack.Navigator에
<Stack.Navigator initialRouteName="Home">
위와 같이 props를 주어서 지정할 수 있다. 이와 같은 경우 처음 사용자가 보게 되는 화면은 HomeScreen 컴포넌트이다.
각 스크린에는 Header의 title을 지정해 줄 수 있다.
<Stack.Screen
name="Home"
component={HomeScreen}
options={{ title: '홈ㅋ' }}
/>
위와 같이 options에 title을 지정해주면
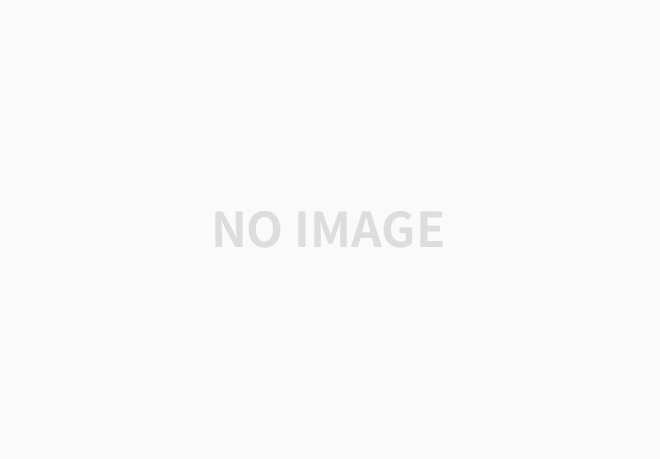
Header의 Title이 변경되는 것을 확인할 수 있다.
이외에 추가적인 props를 넘겨줘야 하는 경우에는
<Stack.Screen name="Home">
{(props) => <HomeScreen {...props} extraData={someData} />}
</Stack.Screen>
이런식으로 사용해주면 된다.
화면 간 이동이 필요한 경우에는 navigation의 navigate 메소드를 사용한다.
import * as React from 'react';
import { Button, View, Text } from 'react-native';
import { NavigationContainer } from '@react-navigation/native';
import { createNativeStackNavigator } from '@react-navigation/native-stack';
function HomeScreen({ navigation }) {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
<Button
title="Go to Details"
onPress={() => navigation.navigate('Details')}
/>
</View>
);
}
// ... other code from the previous section
위와 같이 Button을 만들어준 뒤, onPress에 Screen 이동을 연결한다. navigate 함수가 받는 파라미터는 이동하고자 할 스크린의 name이다.
저장 후 버튼을 클릭해 보면, 스크린이 잘 이동함과 동시에 스택에 새로운 화면이 쌓인다는 것을 확인할 수 있다.
또한 stack에 쌓인 모든 화면들을 clear하고 싶은 경우가 있을 것이다.
이럴 때는,
navigation.popToTop()
navagation의 popToTop() 메소드를 사용해주면 된다.
web에서 쿼리스트링을 통해 params를 사용했다면, RN에서는 아래와 같이 params를 사용할 수 있다.
function HomeScreen({ navigation }) {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Home Screen</Text>
<Button
title="Go to Details"
onPress={() => {
/* 1. Navigate to the Details route with params */
navigation.navigate('Details', {
itemId: 86,
otherParam: 'anything you want here',
});
}}
/>
</View>
);
}
function DetailsScreen({ route, navigation }) {
/* 2. Get the param */
const { itemId, otherParam } = route.params;
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Details Screen</Text>
<Text>itemId: {JSON.stringify(itemId)}</Text>
<Text>otherParam: {JSON.stringify(otherParam)}</Text>
<Button
title="Go to Details... again"
onPress={() =>
navigation.push('Details', {
itemId: Math.floor(Math.random() * 100),
})
}
/>
<Button title="Go to Home" onPress={() => navigation.navigate('Home')} />
<Button title="Go back" onPress={() => navigation.goBack()} />
</View>
);
}
navigate의 두 번째 인자로 params 객체를 전달해주며, 받는 스크린에서는 route객체의 params에 접근하여 꺼내 올 수 있다.
또한 Screen을 나눠줄 때,
<Stack.Screen
name="Details"
component={DetailsScreen}
initialParams={{ itemId: 42 }}
/>
위와 같이 initialParams를 지정해줄 수 있다.
마지막으로, Header에 대한 디자인은
function StackScreen() {
return (
<Stack.Navigator>
<Stack.Screen
name="Home"
component={HomeScreen}
options={{
title: 'My home',
headerStyle: {
backgroundColor: '#f4511e',
},
headerTintColor: '#fff',
headerTitleStyle: {
fontWeight: 'bold',
},
}}
/>
</Stack.Navigator>
);
}
위와 같이 지정해줄 수 있다.
만약 공통된 Header 스타일을 지정해주고 싶다면,
function StackScreen() {
return (
<Stack.Navigator
screenOptions={{
headerStyle: {
backgroundColor: '#f4511e',
},
headerTintColor: '#fff',
headerTitleStyle: {
fontWeight: 'bold',
},
}}
>
<Stack.Screen
name="Home"
component={HomeScreen}
options={{ title: 'My home' }}
/>
</Stack.Navigator>
);
}
Navigator에 props를 넣어준다.
기존에 웹 프론트엔드를 개발 할 때는, 모바일에서 라우팅이 어떤식으로 이루어지는지 궁금했었는데 공식문서를 보며 궁금증이 깔끔하게 해결되었다!
Stack 구조를 사용하지만, 어느정도 Web의 라우팅이랑 비슷한 부분이 많은 것 같다고 생각했고 아직은 낯설지만 차차 적응해 갈 것이라고 생각하고있다.. ㅎㅎ
'React-Native' 카테고리의 다른 글
[RN] 메모리 누수로 인한 IOS 웹뷰 인스턴스 강제종료 (feat. 가비지 컬렉션) (0) | 2025.05.15 |
---|---|
[React Native] RN에서 Webview 사용하기 (0) | 2023.07.19 |
[React Native] Mac 개발환경 세팅 (0) | 2023.07.19 |